As of March 23, 2025, the Python client for Prisma is deprecated and should no longer be used. At the time of writing this, there is no an alternative as Prisma is doing a ground-up rewrite.
SqlAlchemy remains a popular option as an ORM for Python. Alembic can be a good choice for migrations.
I love turning things into services (a bit too much). Sometimes my needs include persistent data, but creating a web backend stack with a database can be a bit of a pain. Because this is such a common task, Abandon Tech maintains a minimalist, but useful FastAPI template that includes:
- FastAPI
- To create the REST API
- Acts as the interface between users and our persistent data
- PostgreSQL
- Persistent data (metrics, user data, etc)
- Ideal for many web applications
- Prisma
- Database migrations and ORM
- Connects our API to the database
- Docker
- Easy dev environment and deployment
- Shareable and reusable environment
FastAPI and Primsa do a lot of heavy lifting and make developing web applications a straight-forward and fun experience.
This is a typical stack for some of the "heavier" web apps I tend to develop--ones with user accounts and other data that needs to be stored indefinitely. If persistent data is not needed, Postgres and Prisma can be removed from the equation for a very lightweight and easy-to-write project.
FastAPI
FastAPI is an incredible, easy to use, well-documented, and fast Python framework that makes writing REST APIs a simple process. The guides they provide on their website are enough for almost any use-case I have ever had. FastAPI also allows projects to be structured flexibly to fit your needs. This allows you to build essentially any web backend you would like completely pain-free.
PostgreSQL
PostgreSQL (Postgres, for short) is a popular relational database that I use as a standard on all of my backend projects. Unless there are specific considerations that would benefit from a noSQL database (or no persistent data is needed at all), I will go with Postgres.
Prisma
Prisma is a very handy Object-Relational Mapping framework that gives us the ability to conveniently write our database schema in a way that is easily read and maintained. We specifically use the Python Prisma client to integrate Prisma with our template.
We include a sample schema with a basic User model example.
Docker
Docker is a hero for hosting and development. Docker Compose allows us to easily spool up the database for this template in both dev and prod, handles importing secrets via a .env
file, and makes configuring and deploying applications in production very straight-forward.
Getting started
To use the template, go ahead and navigate to Github and click Use this template
, then Create a new repository
.
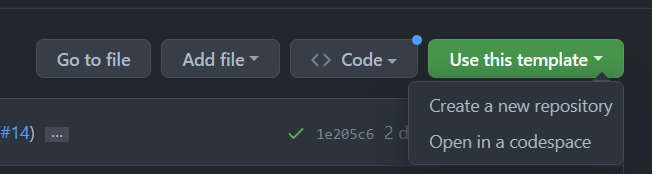
Once you have your own project, there are a few setup steps to get things started.
Create a file named .env
from the sample .env, you should not need to change the contents of the .env
during development and can use the default values.
Optional Setup
If you would like to properly structure your project, feel free to rename the app
directory to match your project name. If you application is named awesome_project
, then convention would be to rename the app
directory to awesome_project
. If you choose to rename this directory, you need to make some configuration changes in a few places.
To start, change the external volume bindings in the docker-compose.yml
to match your new directory naming.
volumes: # mount the source code as a volume, so we can use hot reloading
- "./awesome_project:/app/app:ro"
- "./prisma:/app/prisma:ro"
These lines from the docker-compose file specify that the directory named awesome_project
should be mounted in the container as /app/app
, ro
specifies that the volume should be read-only
.
The new directory also must be changed in the Dockerfile
WORKDIR /app
ADD ./awesome_project ./app
ADD ./prisma ./prisma
ENTRYPOINT ["/bin/bash", "-c"]
CMD ["poetry run prisma db push --schema prisma/schema.prisma && poetry run uvicorn awesome_project:app --host 0.0.0.0 --port 80 $uvicorn_extras"]
Running The Project
Once your project is configured, you can run your app using docker compose. Run the following command in the same directory with the docker-compose.yml
file: docker compose up --build
Test Your Project
Navigate to localhost:8000/docs to view your running test app's Swagger documentation. This is where you can test all of your application's routes.
Now you are ready to go through the FastAPI documentation and Python Prisma Client documentation to create a custom app that fits your needs.
If you have any issues or questions regarding the template, feel free to reach out to us on Github.
Member discussion: